AUCTION
Untuk membuat sistem lelang (Auction) menggunakan empat class, yaitu :
- Person
- Bid
- Lot
- Auction
Berikut adalah souce code yang digunakan :
- Person
/**
* Maintain details of someone who participates in an auction.
* @author Nitama Nurlingga Yotifa.
* @version 20181007
*/
public class Person
{
// The name of this person.
private final String name;
/**
* Create a new person with the given name.
* @param name The person's name.
*/
public Person(String name)
{
this.name = name;
}
/**
* @return The person's name.
*/
public String getName()
{
return name;
}
}
- Bid
/**
* A class that models an auction bid.
* It contains a reference to the Person bidding and the amount bid.
*
* @author Nitama Nurlingga Yotifa.
* @version 20181007
*/
public class Bid
{
// The person making the bid.
private final Person bidder;
// The value of the bid. This could be a large number so
// the long type has been used.
private final long value;
/**
* Create a bid.
* @param bidder Who is bidding for the lot.
* @param value The value of the bid.
*/
public Bid(Person bidder, long value)
{
this.bidder = bidder;
this.value = value;
}
/**
* @return The bidder.
*/
public Person getBidder()
{
return bidder;
}
/**
* @return The value of the bid.
*/
public long getValue()
{
return value;
}
}
- Lot
/**
* A class to model an item (or set of items) in an
* auction: a lot.
*
* @author Nitama Nurlingga Yotifa.
* @version 20181007
*/
public class Lot
{
// A unique identifying number.
private final int number;
// A description of the lot.
private String description;
// The current highest bid for this lot.
private Bid highestBid;
/**
* Construct a Lot, setting its number and description.
* @param number The lot number.
* @param description A description of this lot.
*/
public Lot(int number, String description)
{
this.number = number;
this.description = description;
}
/**
* Attempt to bid for this lot. A successful bid
* must have a value higher than any existing bid.
* @param bid A new bid.
* @return true if successful, false otherwise
*/
public boolean bidFor(Bid bid)
{
if((highestBid == null) ||
(bid.getValue() > highestBid.getValue())) {
// This bid is the best so far.
highestBid = bid;
return true;
}
else {
return false;
}
}
/**
* @return A string representation of this lot's details.
*/
public String toString()
{
String details = number + ": " + description;
if(highestBid != null) {
details += " Bid: " +
highestBid.getValue();
}
else {
details += " (No bid)";
}
return details;
}
/**
* @return The lot's number.
*/
public int getNumber()
{
return number;
}
/**
* @return The lot's description.
*/
public String getDescription()
{
return description;
}
/**
* @return The highest bid for this lot.
* This could be null if there is
* no current bid.
*/
public Bid getHighestBid()
{
return highestBid;
}
}
- Auction
import java.util.ArrayList;
/**
* A simple model of an auction.
* The auction maintains a list of lots of arbitrary length.
*
* @author Nitama Nurlingga Yotifa.
* @version 20181007
*/
public class Auction
{
// The list of Lots in this auction.
private ArrayList<Lot> lots;
// The number that will be given to the next lot entered
// into this auction.
private int nextLotNumber;
/**
* Create a new auction.
*/
public Auction()
{
lots = new ArrayList<Lot>();
nextLotNumber = 1;
}
/**
* Enter a new lot into the auction.
* @param description A description of the lot.
*/
public void enterLot(String description)
{
lots.add(new Lot(nextLotNumber, description));
nextLotNumber++;
}
/**
* Show the full list of lots in this auction.
*/
public void showLots()
{
for(Lot lot : lots) {
System.out.println(lot.toString());
}
}
/**
* Bid for a lot.
* A message indicating whether the bid is successful or not
* is printed.
* @param number The lot number being bid for.
* @param bidder The person bidding for the lot.
* @param value The value of the bid.
*/
public void bidFor(int lotNumber, Person bidder, long value)
{
Lot selectedLot = getLot(lotNumber);
if(selectedLot != null) {
boolean successful = selectedLot.bidFor(new Bid(bidder, value));
if(successful) {
System.out.println("The bid for lot number " +
lotNumber + " was successful.");
}
else {
// Report which bid is higher.
Bid highestBid = selectedLot.getHighestBid();
System.out.println("Lot number: " + lotNumber +
" already has a bid of: " +
highestBid.getValue());
}
}
}
/**
* Return the lot with the given number. Return null
* if a lot with this number does not exist.
* @param lotNumber The number of the lot to return.
*/
public Lot getLot(int lotNumber)
{
if((lotNumber >= 1) && (lotNumber < nextLotNumber)) {
// The number seems to be reasonable.
Lot selectedLot = lots.get(lotNumber - 1);
// Include a confidence check to be sure we have the
// right lot.
if(selectedLot.getNumber() != lotNumber) {
System.out.println("Internal error: Lot number " +
selectedLot.getNumber() +
" was returned instead of " +
lotNumber);
// Don't return an invalid lot.
selectedLot = null;
}
return selectedLot;
}
else {
System.out.println("Lot number: " + lotNumber +
" does not exist.");
return null;
}
}
public void close()
{
System.out.println("Closing auction.");
for(Lot lot : lots)
{
System.out.print(lot.getNumber() + ": " + lot.getDescription());
if ( lot.getHighestBid() == null )
{
System.out.println(" (No bids)");
}
else
{
Bid highestBid = lot.getHighestBid();
System.out.println(" sold to " + highestBid.getBidder().getName() + " for " + highestBid.getValue());
}
}
}
}
Untuk menjalankan program dapat dengan langkah-langkah berikut :
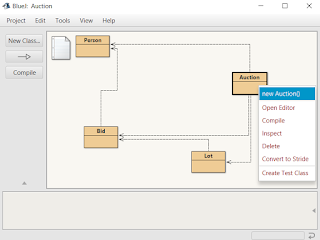 |
Buka New Auction() pada class Auction
|
 |
Pilih enterLot(String description) untuk menambah barang yang akan dilelang
|
 |
Ketik barang-barang yang akan dilelang menggunakan petik dua (string)
|
 |
Buka void show() untuk menampilkan pada terminal window
|
 |
Hasil dari input pada enterLot(String description)
|
 |
Buka new Person(String name) untuk menambahkan nama orang yang
berpartisipasi dalam lelang
|
 |
Buka void bidFor(Number, Person Bidder, long value) untuk input barang, orang,
dan nilai dari suatu barang yang dilelang
|
 |
Jika suatu barang berhasil dilelang maka akan muncul keterangan bahwa lelang berhasil.
Jika tidak, maka akan muncul keterangan bahwa harga yang dimasukkan tidak cukup tinggi
untuk memenangkan lelang.
|
 |
Buka void show() untuk menampilkan pada terminal window
|
 |
Hasil dari lelang pertama
|
 |
Buka void bidFor(Number, Person Bidder, long value) jika ingin melakukan lelang kedua
|
 |
Hasil lelang kedua
|
 |
Buka void close() untuk menutup perlelangan
|
 |
Hasil akhir proses perlelangan |
Komentar
Posting Komentar